Next.js Error Handling: Best Practices Explained (with Examples)
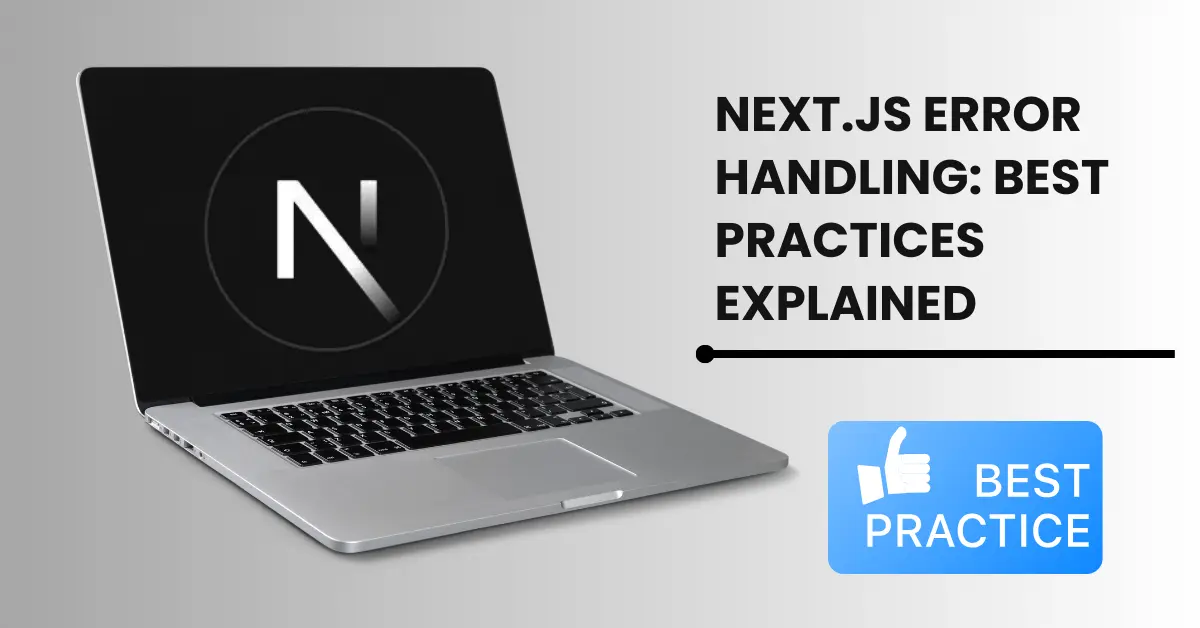
Table Of Content
- Understanding the Problem
- Using Error Boundaries in Next.js
- Step 1: Creating the Error Boundary File
- Step 2: Updating Your Code to Use the Error Boundary
- Step 3: Displaying Friendly Error Messages
- Benefits of Using Error Boundaries
- Error Handling Flow: Step-by-Step Guide
- Best Practices for Error Handling in Nextjs
- Example: Handling Registration Errors
- Backend Validation
- Frontend Handling
- Why Error Boundaries Matter
- Final Thoughts
Error handling is a crucial part of any application, especially when working with frameworks like Next.js. It ensures that users are not faced with unpleasant error messages and that the app runs smoothly under all circumstances. In this article, I’ll walk you through the best practices for error handling in Next.js, with practical steps and examples to improve user experience.
Understanding the Problem
Let's understand with example, when a user tried to register with an already existing email address, the application displayed an ugly and unhelpful error message like this:
Error: User already exists
This type of error message is not user-friendly and results in a poor user experience. A proper error handling mechanism is needed to address this issue and present error messages in a clean, professional manner.
Using Error Boundaries in Next.js
Error boundaries in Next.js allow us to catch errors gracefully and display appropriate messages without breaking the app. Here's how you can implement error boundaries step by step.
Step 1: Creating the Error Boundary File
- Create a New File: Inside the
app
directory, create a file namederror-boundary.js
. This file will contain the logic for handling errors. - Set Up the Component: Start by defining an error boundary component. Here’s an example:
'use client';
import React from 'react';
export default function ErrorBoundary({ error }) {
return (
<div style={{ padding: '20px', textAlign: 'center' }}>
<h2>Something went wrong</h2>
<p>{error.message || 'An unexpected error occurred.'}</p>
</div>
);
}
The use client
directive ensures that this component runs on the client side.
Step 2: Updating Your Code to Use the Error Boundary
To use the error boundary:
- Import the
ErrorBoundary
component wherever needed. - Wrap parts of your UI prone to errors with this boundary.
For instance:
import ErrorBoundary from './error-boundary';
export default function RegisterPage() {
return (
<ErrorBoundary>
{/* Registration form or other components */}
</ErrorBoundary>
);
}
Now, whenever an error occurs, the error boundary will catch it and display a friendly error message.
Step 3: Displaying Friendly Error Messages
Instead of showing raw error messages like "User already exists," we can customize the output for better user experience:
if (error.code === 'USER_EXISTS') {
return <p>User already exists. Please use a different email address.</p>;
}
This approach provides clarity and prevents confusion for end-users.
Benefits of Using Error Boundaries
Using error boundaries in Next.js comes with several advantages:
Benefit | Explanation |
---|---|
Separation of Concern | Keeps error-handling logic separate from the main application logic. |
Enhanced User Experience | Prevents crashes and provides clear, actionable error messages. |
Maintainability | Simplifies future updates and changes to error-handling strategies. |
Error Handling Flow: Step-by-Step Guide
Here’s how the error handling process works in our application:
- User Action: The user attempts to register with an already existing email address.
- Error Triggered: The application throws an error.
- Error Boundary Activates: The error boundary component catches the error.
- User-Friendly Message Displayed: The component displays a clean error message to the user.
Best Practices for Error Handling in Nextjs
To ensure your application is robust and user-friendly, follow these best practices:
- Use Descriptive Error Messages: Avoid generic error messages. Be specific and informative.
- Log Errors for Debugging: Use a logging service like Sentry to keep track of errors for future debugging.
- Provide Actionable Feedback: Whenever possible, suggest steps the user can take to resolve the issue.
- Test Error Scenarios: Simulate potential errors during development to verify that the handling logic works as expected.
- Fallback Components: Always have a fallback UI for unexpected errors, ensuring a smooth user experience.
Example: Handling Registration Errors
Below is a sample implementation for handling errors during user registration.
Backend Validation
Ensure the backend returns proper error codes. For example:
if (emailExists) {
throw new Error('USER_EXISTS');
}
Frontend Handling
On the frontend, handle the error like this:
try {
await registerUser(data);
} catch (error) {
if (error.message === 'USER_EXISTS') {
setError('This email is already registered. Try logging in instead.');
} else {
setError('An unexpected error occurred. Please try again.');
}
}
Why Error Boundaries Matter
Without error boundaries, any uncaught error can crash your app or result in a poor user experience. Here’s what error boundaries solve:
- Prevents App Crashes: Ensures the rest of the app remains functional even if one part fails.
- Improves Error Visibility: Makes it easier to identify where the error occurred.
- Enhances Professionalism: Shows users that you care about their experience.
Final Thoughts
Implementing proper error handling is essential for building reliable applications. Next.js provides the tools you need to handle errors gracefully, ensuring a smooth experience for your users. By following the steps outlined above, you can make your application more robust and user-friendly.