Handling Errors in Next.js 14: A Step-by-Step Guide
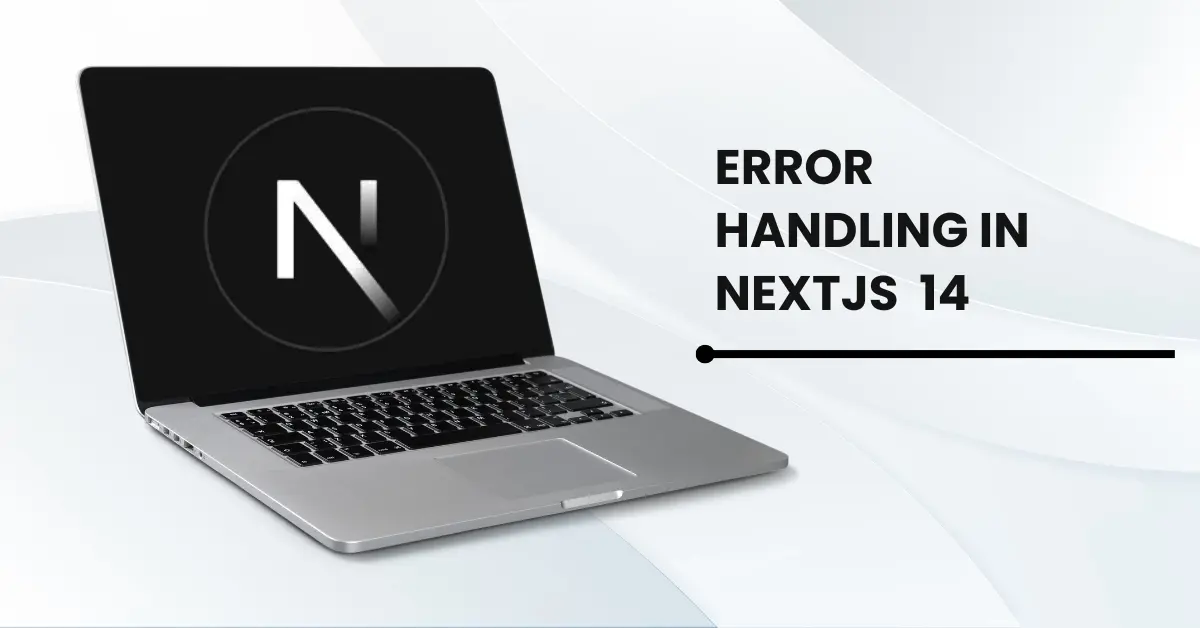
Table Of Content
- Introduction to Error Handling in Next.js
- Step 1: Creating the `error.js` File
- Step 2: Understanding How `error.js` Works
- Step 3: Throwing Errors in `page.js`
- Step 4: Accessing the Error Message in `error.js`
- Step 5: Wrapping Multiple Components with Error Boundaries
- Wrapping Individual Components
- Global Error Boundary
- Step 6: Testing in Production
- Conclusion
In this tutorial, I’ll walk you through how to handle errors effectively in Next.js 14. Error handling in Next.js involves creating a specialized error boundary component that catches and displays errors in a user-friendly manner. Let’s dive into the process step-by-step.
Introduction to Error Handling in Next.js
Error handling in Next.js works similarly to the layout.js
and loading.js
components. By following specific naming conventions, we can easily implement an error boundary for our application.
Step 1: Creating the error.js
File
The first step is to create a new file named error.js
. This file acts as the error boundary component and is responsible for catching and displaying errors.
-
Create the file:
Inside your component folder, create a new file namederror.js
. -
Declare it as a client component:
Add the directiveuse client
at the top of the file, as the error component must be a client component."use client";
-
Export the error component:
Use therafce
snippet (React Arrow Function Component Export) to quickly scaffold the component. This component will handle errors and optionally provide functionality to reset the page.export default function ErrorComponent({ error, reset }) { return ( <div> <p>{error.message}</p> <button onClick={reset}>Reset</button> </div> ); }
Step 2: Understanding How error.js
Works
When you add an error.js
file to your component folder, it automatically wraps the corresponding page in an error boundary. This means that if an error occurs in your page.js
file, the error.js
component will display the error instead.
Step 3: Throwing Errors in page.js
To see the error boundary in action, let’s simulate an API request failure in the page.js
file.
-
Check the API response:
Add a condition to check if the response is not OK.const response = await fetch("/api/some-endpoint"); if (!response.ok) { throw new Error("Failed API request"); }
-
Simulate an error:
Refresh the page, and the error boundary component will display the message "Failed API request".
Step 4: Accessing the Error Message in error.js
To display the error message dynamically, update the error.js
file:
-
Replace the static content with the
error.message
property.<p>{error.message}</p>
-
Add a reset button to reattempt the failed request. Use the
reset
function provided to the component.<button onClick={reset}>Try Again</button>
Step 5: Wrapping Multiple Components with Error Boundaries
You can extend error handling to wrap multiple components or even the entire application. Here’s how:
Wrapping Individual Components
Move the error.js
file to the layout.js
level of a folder. This wraps all child components (e.g., bar
, counter
, prisma
) in the error boundary.
Global Error Boundary
To create a global error boundary, add a root-level error.js
file in the app
folder. This component wraps the entire application and serves as a fallback for any uncaught errors.
Step 6: Testing in Production
While the custom error boundary works in development mode, some features, like global error handling, can only be fully tested in production. To test:
- Deploy the application to a production environment.
- Trigger an error to see your custom error component in action.
Conclusion
With the steps outlined above, you can handle errors effectively in your Next.js 14 application. Whether it's wrapping individual components or creating a global error boundary, these techniques ensure a smoother user experience in the face of unexpected errors.