SEO in Next.js: Optimize Your Website for Search Engines
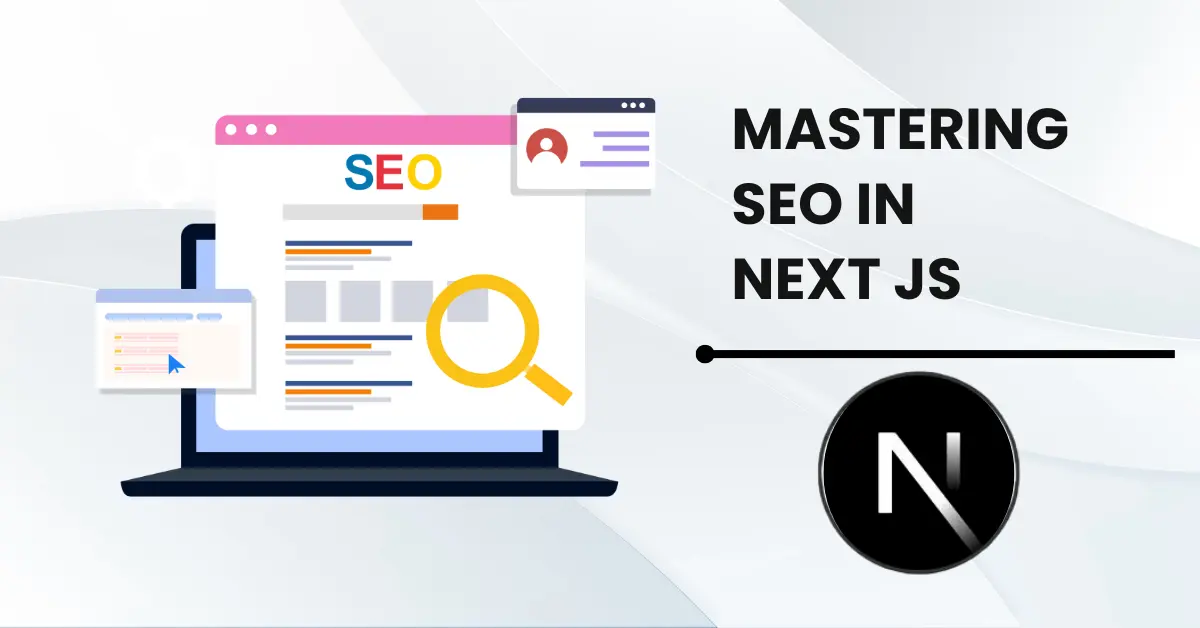
Table Of Content
- Why SEO Is Essential for Your Next.js App
- Step 1: Set Up a Configuration File
- Step 2: Create an SEO Utility File
- Step 3: Integrate SEO Metadata in Root Layout
- Step 4: Enhance with Rich Snippets
- Step 5: Optimize Static Pages
- Step 6: Handle Dynamic Pages with Generate Metadata
- Step 7: Verify Your Domain in Google Search Console
- Step 8: Create a Blog Section
- Blog Structure
- Step 9: Style Your Blog
- Step 10: Optimize URL Structure
- Step 11: Regularly Update and Index
- Summary
Hello everyone, it's Sonu here. In this guide, I’ll walk you through optimizing your Next.js app for search engines. I'll keep the steps simple and actionable, so you can implement them quickly and effectively.
Why SEO Is Essential for Your Next.js App
- Increased Visibility: A well-optimized website ranks higher on search engines like Google.
- More Users, No Extra Cost: SEO drives organic traffic without additional marketing expenses.
- Ease of Access: Users can find your site easily, explore your content, and engage with your product.
Now, let’s look at how you can achieve this in your Next.js app.
Step 1: Set Up a Configuration File
Start by creating a config.ts
file to store constant values. This file can include:
- Domain name
- App name
- App description
This makes it easy to update these details later without hunting through your codebase.
Step 2: Create an SEO Utility File
Add an seo.tsx
file to your project in a utility folder. This file will house two key functions:
-
Get SEO Tags
This function imports metadata from Next.js' built-inmetadata
object, giving access to various meta tags, such as:- Title
- Description
- Keywords
- Application name
- Open Graph and Twitter metadata
- Canonical URL
-
Render Schema Tags
Use this function to create structured data for rich snippets, enhancing the way your site appears in search results.
Example Metadata Configuration:
export const getSeoTags = () => ({
title: "Your App Title",
description: "Your App Description",
keywords: "keyword1, keyword2, keyword3",
applicationName: "Your App Name",
openGraph: { ... },
twitter: { ... },
canonical: "https://yourwebsite.com",
});
Step 3: Integrate SEO Metadata in Root Layout
In your root-layout.tsx
file:
- Import the SEO functions.
- Add them to the layout to ensure all pages inherit the base metadata.
This serves as the foundation for your site’s SEO.
Step 4: Enhance with Rich Snippets
Rich snippets provide additional information in search results. For example, you can:
- Add educational content ratings.
- Include product offers or plans.
Insert the schema directly into the root layout or specific pages to enhance search visibility.
Example Rich Snippet Code:
const renderSchemaTags = () => (
<script type="application/ld+json">
{JSON.stringify({
"@context": "https://schema.org",
"@type": "EducationalApplication",
name: "Your App Name",
ratingValue: "4.5",
reviewCount: "150",
})}
</script>
);
Step 5: Optimize Static Pages
For static pages like "About" or "Contact," define unique metadata:
- Title: Page-specific title
- Description: Custom description
- Canonical URL: Unique URL for the page
Update these directly in each static page’s metadata section for better results.
Step 6: Handle Dynamic Pages with Generate Metadata
Dynamic pages, such as blog posts or products, require dynamic metadata. Use Next.js’ generateMetadata
feature to achieve this.
Steps:
- Export a
generateMetadata
function. - Fetch the necessary data dynamically.
- Return metadata specific to each page or article.
Example Code:
export async function generateMetadata({ params }) {
const article = await getArticleData(params.slug);
return {
title: article.title,
description: article.description,
openGraph: { ... },
};
}
This ensures every article has unique metadata, improving SEO for individual pages.
Step 7: Verify Your Domain in Google Search Console
To help Google crawl your site effectively:
- Visit Google Search Console.
- Add your domain and verify ownership by adding meta tags or DNS records.
- Use the URL Inspection Tool to request indexing for new pages.
Step 8: Create a Blog Section
Blogs are excellent for driving organic traffic. Here's how to set up a blog in your Next.js app:
Blog Structure
- Header Link: Add a “Blog” link in the site header.
- Simple Cards: Display articles with a title, category, and brief description.
- Dynamic Pages: Each article has its own page with dynamic metadata.
Directory Layout:
/components
: Contains reusable components likeAvatar
,Badge
, andCard
./pages/blog
: Houses the main blog page./pages/blog/[slug]
: Handles individual article pages.
Content Management:
Store article data in a content.ts
file as an array of objects for smaller projects. Example structure:
export const articles = [
{
slug: "seo-in-nextjs",
title: "SEO in Next.js",
description: "A guide to optimizing your Next.js site.",
author: "Artam",
category: "SEO",
},
];
Step 9: Style Your Blog
Use Next.js’ public
folder for storing assets, or organize them in a dedicated assets
directory. Define a consistent style for:
- Headings: H2, H3, etc.
- Paragraphs
- Image Cards
Step 10: Optimize URL Structure
Ensure your blog URLs are simple and descriptive:
- Good:
https://yourwebsite.com/blog/seo-in-nextjs
- Avoid:
https://yourwebsite.com/blog/12345
Use the slug
field from your article data for this purpose.
Step 11: Regularly Update and Index
Whenever you add new blog posts or pages:
- Go to Google Search Console.
- Use the URL Inspection Tool to request indexing.
This speeds up the process of appearing in search results.
Summary
In this guide, we covered:
- Setting up SEO in Next.js
- Adding metadata for static and dynamic pages
- Using rich snippets for enhanced search results
- Creating and optimizing a blog section
- Leveraging Google Search Console for indexing
By implementing these steps, you can make your Next.js site highly optimized for search engines. Start applying these techniques today to boost your website's performance!