Fix Common mistakes With Nextjs App Router
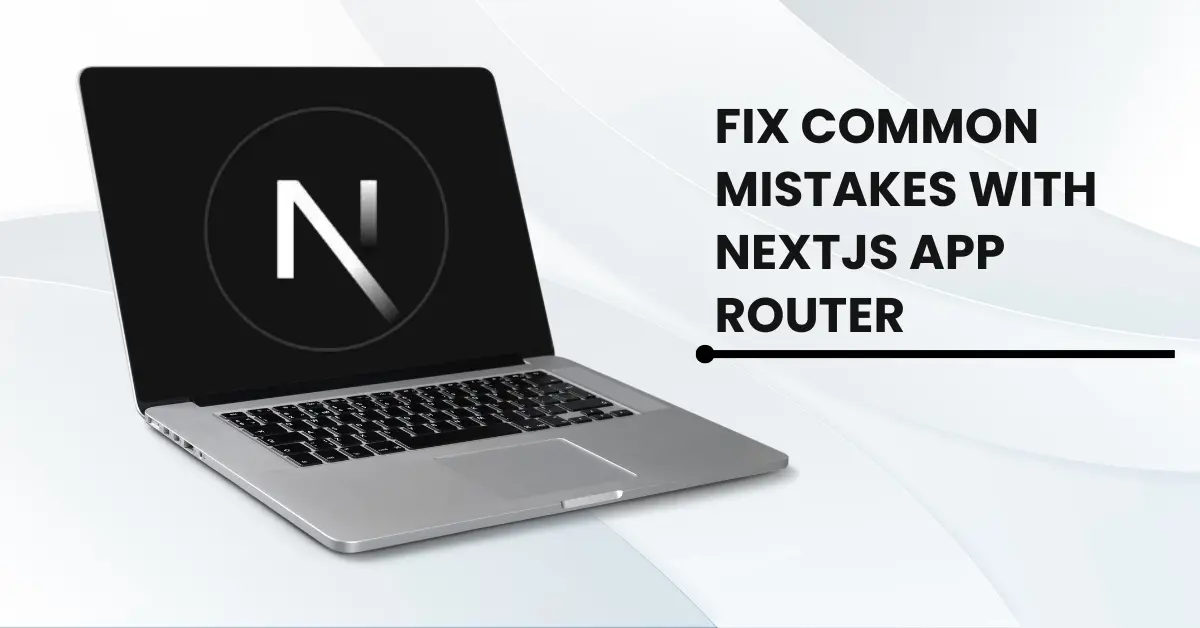
Table Of Content
- 1. Calling Route Handlers from Server Components
- 2. Misunderstanding Static and Dynamic Route Handlers
- Example Table: Static vs Dynamic Route Handlers
- 3. Misusing Route Handlers with Client Components
- 4. Ignoring the Power of Static Export
- Steps to Use Static Export:
- 5. Overcomplicating Webhook Implementations
- 6. Overlooking Built-In Helper Functions
- 7. Forgetting to Optimize API Routes for Caching
- 8. Not Utilizing Middleware Correctly
- 9. Overcomplicating Dynamic Routes
- 10. Neglecting Error Handling in Route Handlers
- Final Thoughts
As I have reviewed countless repositories and spoken with hundreds of developers working with the Next.js App Router, I've identified some common pitfalls. Avoiding these mistakes can significantly improve your Next.js applications. Let's break them down step by step.
1. Calling Route Handlers from Server Components
Many developers mistakenly believe they need to call route handlers directly from server components. Here's why this is unnecessary and how you can simplify your approach:
-
What is a Route Handler?
- A route handler allows the use of HTTP verbs like
GET
,POST
,PUT
, andDELETE
to expose APIs for fetching or mutating data.
- A route handler allows the use of HTTP verbs like
-
The Common Mistake:
- Developers create a route handler that returns JSON, then fetch it inside a React server component using a hardcoded URL.
- Example:
const data = await fetch("http://localhost:3000/api");
-
Why It's a Problem:
- Hardcoding URLs introduces unnecessary complexity.
- It creates an extra network request even when both the server and client run on the same environment.
-
Better Approach:
- Instead of fetching through the route handler, directly call the required function in your server component.
const data = await getUserData(); // Call the function directly
- Instead of fetching through the route handler, directly call the required function in your server component.
By removing the middleman, your application becomes more efficient and easier to maintain.
2. Misunderstanding Static and Dynamic Route Handlers
Route handlers in Next.js can be static or dynamic by default. Misunderstanding these settings can lead to unintended behaviors.
-
Example Scenario:
- A route handler generates a current timestamp using
new Date()
:export default function handler(req, res) { res.json({ date: new Date().toLocaleString() }); }
- A route handler generates a current timestamp using
-
Observed Behavior:
- During local development (
next dev
), the timestamp updates with every request. - After production build (
next build
), the output is cached and remains static.
- During local development (
-
Key Takeaways:
- By default, route handlers in production behave as cached static pages.
- To make a handler dynamic, you must opt-in by:
- Accessing
req
parameters or headers. - Using dynamic HTTP methods like
POST
.
- Accessing
Example Table: Static vs Dynamic Route Handlers
Behavior | Static | Dynamic |
---|---|---|
Default Setting | Cached responses | Real-time processing |
HTTP Methods | GET (default behavior) | POST , or using req for dynamic input |
Common Use Cases | Pre-rendered data, static exports | Webhooks, real-time updates |
3. Misusing Route Handlers with Client Components
Client components often lead developers to overuse route handlers. Here's why you might not need them:
-
The Misconception:
- Developers use route handlers to fetch data required by client components, assuming this is the only way.
-
Better Solution:
- Use server-side rendering (SSR) or static site generation (SSG) to fetch data and pass it as props to client components. This eliminates the need for additional route handlers.
4. Ignoring the Power of Static Export
Static export is a feature that many overlook but can be extremely beneficial. With it, you can generate static files for deployment anywhere, such as:
- S3 buckets
- Content delivery networks (CDNs)
Steps to Use Static Export:
- Add
next.config.js
:module.exports = { output: "export", };
- Run the build command:
next build && next export
- Deploy the generated files to your preferred static hosting platform.
5. Overcomplicating Webhook Implementations
When implementing webhooks, developers often create unnecessary complexity by adding multiple layers of abstraction.
- Simpler Alternative:
- Directly handle webhook requests within a route handler using
POST
.export async function POST(req) { const body = await req.json(); // Process webhook data return new Response("Success", { status: 200 }); }
- Directly handle webhook requests within a route handler using
6. Overlooking Built-In Helper Functions
Next.js provides helper functions like cookies()
and headers()
, yet many developers write custom parsers unnecessarily.
-
Example Usage:
export async function GET() { const userCookies = cookies(); const userHeaders = headers(); return new Response(JSON.stringify({ userCookies, userHeaders })); }
-
These helpers simplify reading request data, reducing boilerplate code.
7. Forgetting to Optimize API Routes for Caching
API routes can be optimized for caching to improve performance:
-
Default Behavior:
- Static by default for
GET
requests.
- Static by default for
-
To Enable Dynamic Caching:
- Use HTTP cache headers:
export async function GET() { const data = await fetchExternalData(); return new Response(JSON.stringify(data), { headers: { "Cache-Control": "no-store", // Disables caching }, }); }
- Use HTTP cache headers:
8. Not Utilizing Middleware Correctly
Middleware in Next.js can handle tasks like authentication and logging before reaching the route handlers.
- Example:
export function middleware(req) { const token = req.headers.get("Authorization"); if (!token) { return new Response("Unauthorized", { status: 401 }); } }
This approach reduces redundancy across route handlers.
9. Overcomplicating Dynamic Routes
Dynamic routes in Next.js allow flexibility but can lead to unnecessary complexity if not implemented correctly.
- Simplified Approach:
- Use
getStaticPaths
andgetStaticProps
for static generation. - Example:
export async function getStaticPaths() { return { paths: [{ params: { id: "1" } }], fallback: false, }; } export async function getStaticProps({ params }) { const data = await fetchData(params.id); return { props: { data } }; }
- Use
10. Neglecting Error Handling in Route Handlers
Error handling is often overlooked, leading to silent failures. Here's how to handle errors effectively:
- Example:
export async function GET(req) { try { const data = await fetchData(); return new Response(JSON.stringify(data)); } catch (error) { return new Response("Error fetching data", { status: 500 }); } }
Proper error handling ensures a better user experience and easier debugging.
Final Thoughts
By avoiding these common mistakes and following best practices, you can build robust and efficient Next.js applications. Stay curious and keep refining your approach as you explore the capabilities of the Next.js App Router.